A
Router
is commonly known as a hardware device for computer networking, we all have wired or wireless routers at home, offices or other locations for devices networking.
Router in Web Application
We'll get different page content from a site when we change the string after the domain name in a URL, or click the menu items of a webpage. This navigation feature of web apps is done by a Router
middleware of the modern web app as well. Accessing to a specific URL path will trigger a respective route
response from the web app's routing table
, the web app usually uses a specific Router middleware to manage all the routes
, no matter whether the routing pages are rendered from server-side or client-side. For example:
- Backend routing: in
Node.js
, we can implement routes byexpress.js
, and eachendpoint
will render thetemplate
to html for client-side, each time accessing to a new path(route), there will be a new request sent to the backend.
const express = require('express'); let app = express(); app.listen(8080); let router = express.Router(); // endpoints router.get('/amazon', (req, res) => { res.render(<code>amazon</code>)}); router.get('/discovery', (req, res) => { res.render(<code>discovery</code>)});
- Front-end routing: React.js and Vue.js have their own favorable routing middleware from their ecosystem:
react-router
andvue-router
respectively. The front-end routing is always used forSPA
(single page application) website, as it prevents un-neccessary requests to the server, the change of a route accessing will lead to only partial internal view change inside the page, which is usually related to a specific component view of the web app, and whole page rendering is avoided.
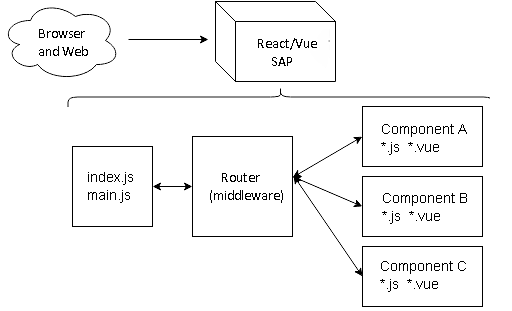
Understanding Routing mechanism in SPA by Analogy
We don't care whether it is called as a path, a uri, or a route when browsing different pages of the web app, however, we need to have a clear understanding of the router and when we implement it when developing. It takes some time to switch the hardaware concepts to things we do in web development. I have a better understanding of these Router terms in web dev by analogizing them with the use of a wired Router in the office networking senario, the main points are listed in the following table.
Use Router in React.js
React Router: the latest release version will be v6 https://github.com/ReactTraining/react-router/releases
npm install react-router
v5 code:
// ./components/Application.js import React from 'react'; import { Link, Route, Switch} from 'react-router-dom'; import Discovery from './components/Discovery'; import Amz from './components/Amazon'; export default function Navigation() { return ( <div> <ul> <li><Link to="/amazon">Amazon</Link></li> <li><Link to="/discovery">Discovery</Link></li> </ul> {/* Routing Table */} <Switch> <Route path="/amazon" component={<Amazon />} /> <Route exact path="/discovery" component={<Discovery />} /> </Switch> </div> ); }
// App.js import React from "react"; import ReactDOM from "react-dom"; import { BrowserRouter as Router} from 'react-router-dom'; import Application from './components/Application.js'; ReactDOM.render(( <Router> <Application /> </Router> ), document.getElementById("root"));
Use Router in Vue.js
npm install vue-router
// App.vue (View) <template> <div id="app"> <div id="nav"> <!-- you connect cables to the router ports according to the definition in routing table: router-link to will change the conent inside the tag into an a tag with href link --> <router-link to="/discovery">Discovery</router-link> <router-link to="/amazon">Amazon</router-link> </div> <!-- display routes matched components here, put this where your components pages/views shows --> <router-view/> </div> </template>
// router.js (Router) import VueRouter from 'vue-router' // you buy a router device Vue.use(VueRouter) // you open the box and connect it to power // the computers your router will manage import Amazon from "./components/Amazon.vue"; import Discovery from "./components/Discovery.vue"; // you power on the router and setup routing table with routes mannually let routes = new VueRouter({ routes_table: [ // route for port 1: { path: "/amazon", // an MAC or IP address component: Amazon, // a computer name }, // route for port 2: { path: "/discovery", // an ip address component: Discovery, // a computer name }, ]; }); // initialize a router with routing table const router = new VueRouter({ routes } export default router
// main.js import Vue from 'vue' import App from './App.vue' import router from './router' new Vue({ router, // connect router to ISP(the SPA) render: h => h(App) }).$mount('#app')
Conclusion
- The Routing mechanism of web app pages navigation is very simillar to the application of a hardware Router in a office/home networking secnario.
- The way to use the Router middleware in React and Vue frontend framework is also simillar.
Comments